Advanced C Programming
You will gain a deep understanding of pointers, memory allocation, and advanced concepts like multithreading and network programming.
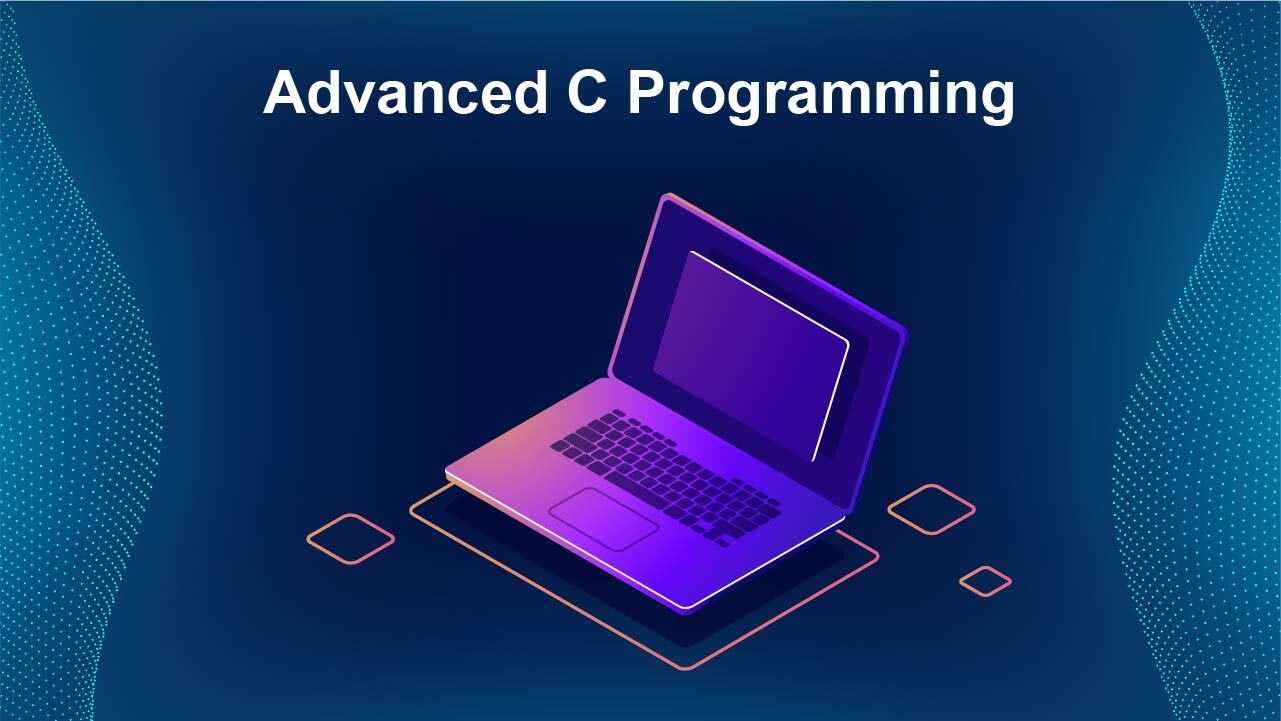
Course Information
-
Module 1
Getting Started (00:14:21)
Types of C variables (00:09:35)
First C program (00:15:27)
printf() and its Purpose (00:15:16)
Receiving Inputs (00:07:12)
Example 1 (00:08:10)
Example 2 (00:11:40)
Example 3 (00:10:37)
Instructions and Operators (00:06:15)
Arithmetic Instructions (00:16:54)
Type Conversion (00:08:45)
Hierarchy of Operations (00:12:12)
Example 1 (00:08:44)
Example 2 (00:15:24)
Variables and datatypes Concept (00:02:12)
Module 1 test (00:00:00)
Assignment Module1 (00:00:00)
-
Module 2
If-else construct (00:11:53)
if-else statement (00:12:28)
Example 1 (00:11:23)
Example 2 (00:14:02)
Example 3 (00:15:15)
else if statement and operators (00:13:44)
else if statement and operators (Contd) (00:15:52)
else if statement and operators (Contd) (00:09:37)
else if statement Examples (00:13:41)
else if statement Example 2 (00:11:20)
else if statement Example 3 (00:09:26)
else if statement Example 4 (00:13:07)
switch case statement (00:10:41)
switch case statement (Contd) (00:12:16)
switch case statement Example 1 (00:06:45)
switch case statement Example 2 (00:02:20)
switch case statement Example 3 (00:11:32)
switch case statement Summary (00:01:48)
while loop (00:07:52)
while loop (Contd) (00:10:31)
while loop Example 1 (00:09:18)
while loop Example 2 (00:08:28)
while loop Example 3 (00:14:27)
while loop Example 4 (00:07:48)
while loop Summary (00:02:36)
for loop and do while loop (00:07:48)
for loop and do while loop (Contd) (00:13:57)
Nested Loops (00:04:43)
Multiple statements in for (00:18:06)
do - while loop (00:04:32)
Example 1 (00:12:20)
Example 2 (00:13:34)
Example 3 (00:06:52)
Summary (00:02:18)
For While-Do While Concept (00:02:18)
if else Concept (00:02:35)
Module 2 Test (00:00:00)
Assignment Module2 (00:00:00)
-
Module 3
Functions (00:07:34)
Functions (Contd) (00:09:17)
Passing values to fucntions (00:18:07)
Scope rule in functions (00:07:06)
Using Library Functions (00:09:53)
Functions Example 1 (00:12:12)
Functions Example 2 (00:09:26)
Functions Summary (00:01:47)
Pointers (00:10:58)
Pointer Notation (00:14:15)
Function Calls (00:08:14)
Application of Function calls (00:05:46)
Example 1 (00:06:30)
Example 2 (00:07:03)
Example 3 (00:07:08)
Example 4 (00:07:12)
Summary (00:02:27)
Recursion (00:10:41)
Recursion and Stack (00:11:10)
Example 1 (00:08:30)
Example 2 (00:07:34)
Example 3 (00:19:29)
Pointers Concept (00:01:39)
Functions Concept (00:02:26)
Recursion Concept (00:02:16)
Test (00:00:00)
Assignment Module3 (00:00:00)
-
Module 4
What are Arrays ? (00:14:43)
More about Arrays (00:14:35)
Arrays Example 1 (00:02:35)
Arrays Example 2 (00:04:52)
Arrays Example 3 (00:05:02)
Arrays Example 4 (00:10:02)
Arrays Example 5 (00:08:21)
Pointers and Arrays (00:17:31)
Pointers and Arrays (Contd) (00:05:23)
Arrays to a function (00:06:58)
Arrays Example 1 (00:02:47)
Arrays example 2 (00:03:58)
Arrays example 3 (00:05:59)
Arrays Example 4 (00:04:59)
Summary (00:03:03)
Two - Dimensional Array (00:09:19)
Pointers and Two - Dimensional Arrays (00:06:00)
Pointers to an Array (00:11:22)
Array of Pointers (00:06:39)
Arrays Example 1 (00:03:22)
Arrays Example 2 (00:04:26)
Arrays Example 3 (00:07:13)
Arrays Example 4 (00:05:41)
Summary (00:01:31)
Strings (00:16:07)
Pointers and strings (00:15:26)
Example 1 (00:10:12)
Example 2 (00:08:03)
Example 3 (00:09:41)
Example 4 (00:11:19)
Summary (00:02:04)
Multiple Strings (00:05:34)
Array of pointers to strings (00:05:21)
Limitations of array of pointers to strings (00:08:57)
Example 1 (00:06:08)
Example 2 (00:12:06)
Example 3 (00:09:33)
Summary (00:02:14)
Arrays Concept (00:03:10)
Strings Concept (00:01:49)
Module 4 Test (00:00:00)
Assignment Module4 (00:00:00)
-
Module 5
Structures (00:04:17)
Declaring a structure (00:08:49)
Storing Structured Elements (00:04:16)
Array of Structures (00:11:59)
Additional features of structures (00:09:06)
Example 1 (00:02:27)
Example 2 (00:03:16)
Example 3 (00:14:36)
Example 4 (00:18:41)
Summary (00:02:46)
Structures Concept (00:02:06)
Module 5 Test (00:00:00)
Assignment Module5 (00:00:00)
-
Module 6
Console I/O (00:03:28)
Formatted Console I/O Functions (00:10:51)
Escape Sequences (00:08:54)
Unformatted Console I/O Functions (00:04:03)
gets() and puts() (00:05:26)
Example 1 (00:06:03)
File I/O (00:02:38)
File Operations (00:15:12)
String (Line) I/O in files (00:08:49)
Example 1 (00:09:54)
Example 2 (00:12:21)
Summary (00:01:39)
Typecasting (00:08:29)
Macro Expansion (00:04:53)
File Inclusion (00:07:12)
Using argc and argv (00:04:11)
Mini project (00:07:10)
Mini project (Contd) (00:13:23)
Mini project (Contd) (00:20:49)
Summary (00:05:30)
Module 6 Test (00:00:00)
Assignment Module6 (00:00:00)
-
Module 7
DSA Intro (00:17:07)
stacks (00:20:52)
Queue (00:46:13)
Linked List (00:47:59)
CLL (00:41:23)
DLL (00:40:23)
Tree (00:35:04)
bst1 (00:56:30)
Heap (00:36:22)
Hash (00:31:28)
Graph1 (00:58:26)
Graph 2 (00:41:07)
BFS (00:40:46)
DFS (00:36:09)
Shortest Path (01:27:56)
MST1 (00:51:07)
APSP (00:27:20)
Sort (01:18:15)
Sort-1 (00:28:27)
ASYMP (00:34:19)
Data Structure Concept (00:02:00)
Graphs Concept (00:01:45)
Hashing Concept (00:02:00)
Heaps Concept (00:02:10)
Linked List Concept (00:01:50)
Queues Concept (00:01:30)
Searching and Sorting Concept (00:01:54)
Stacks Concept (00:01:25)
Trees Concept (00:01:57)
Module 7 Test (00:00:00)
Assignment Module7 (00:00:00)
-
Final Certification Examination
Final Test (00:00:00)
Course Content
Authors
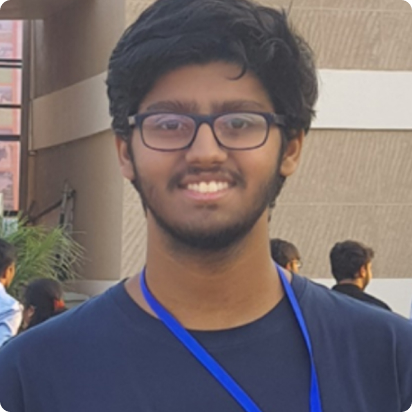
Vivek Pillai
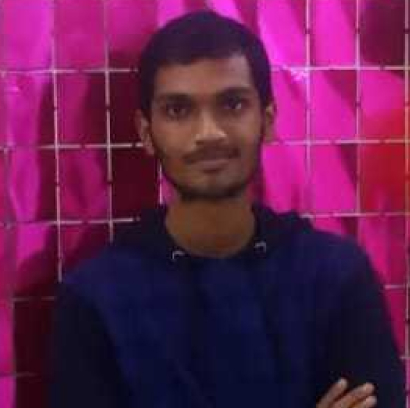
Aditya sujeet Zanjurne
15 DAYS ACCESS
₹0
Start learning today! Switch to full course access, when satisfied with the trial.
PROJECT
Data Modeling with Postgres
In this project, you’ll model user activity data for a music streaming app called Sparkify. You’ll create a relational database and ETL pipeline designed to optimize queries for understanding what songs users are listening to. In PostgreSQL you will also define Fact and Dimension tables and insert data into your new tables.