Java Programming
You will learn the fundamentals of Java programming, including object-oriented programming concepts, data structures, algorithms, and design patterns.
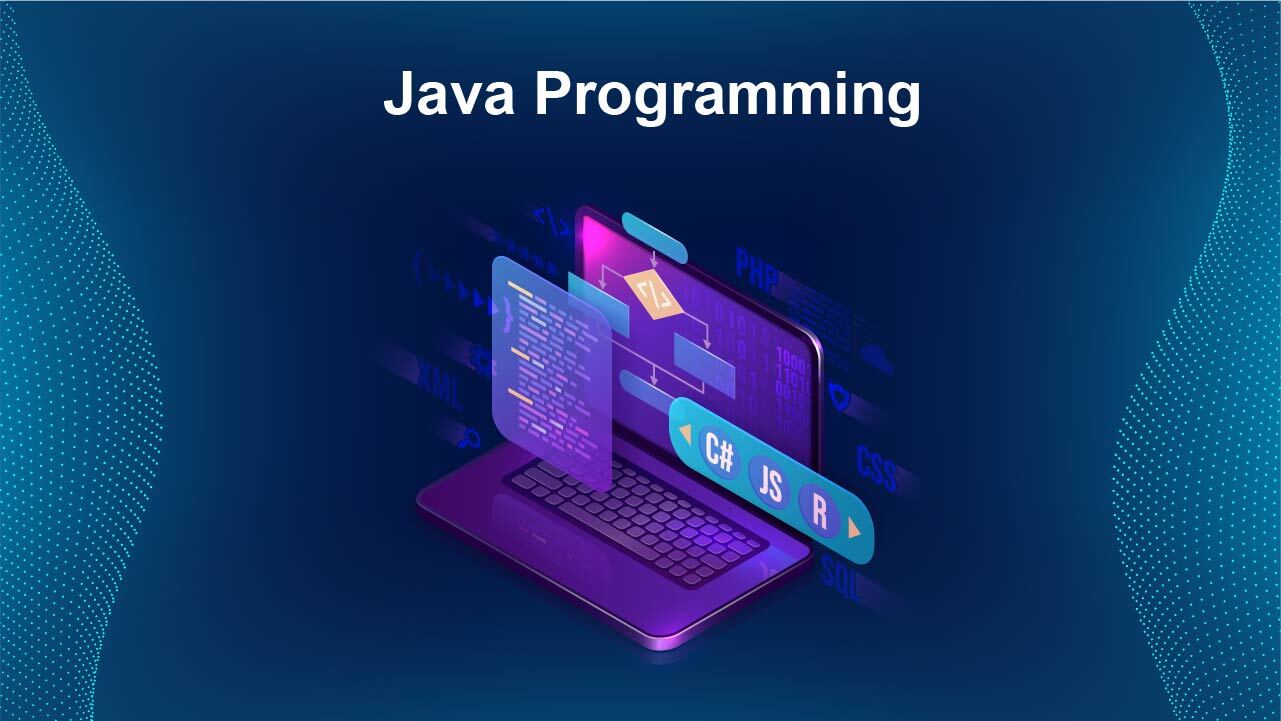
Course Information
-
Module-1
Overview1 (00:06:43)
Overview2 (00:11:14)
Java history (00:02:03)
Features (00:10:10)
JDK_JRE_JVM (00:08:56)
Installing Java (00:03:41)
Running Hello World (00:05:31)
Data types (concept) (00:01:50)
Range of Datatypes (00:04:29)
Variables (00:17:57)
Basic Input/Output (00:10:33)
Operators (00:11:04)
Number System (00:11:31)
Typecast (00:09:40)
Static Keywords (Concept) (00:01:24)
Keywords (00:03:44)
Number Conversion Exercise (00:10:09)
Left shift-Right shift (00:08:05)
Increment Decrement (00:09:24)
Bitwise Operator (00:07:43)
Condition1 (00:08:42)
Condition2 (00:13:34)
Foreach loop (00:05:20)
Loops1 (00:10:26)
Loops2 (00:19:53)
Conditions (Concept) (00:02:06)
Dataflow & Controlflow1 (00:12:03)
Dataflow & Controlflow2 (00:10:12)
Dataflow & Controlflow3 (00:22:07)
Dataflow & Controlflow4 (00:10:00)
Array1 (00:26:08)
Array2 (00:22:59)
Array3 (00:20:18)
Array4 (00:09:11)
Command line args (00:05:58)
Module1 test (00:00:00)
Maths (00:08:38)
Assignment Module1 (00:00:00)
-
Module-2
guessgame1 (00:15:11)
guessgame2 (00:06:33)
Function-Java method (Concept) (00:01:48)
Lambda functions Concept (00:01:57)
Methods (00:23:01)
Recursion (Concept) (00:01:15)
Recursion (00:14:26)
String (00:18:53)
String demo (00:27:47)
Test (00:00:00)
Assignment Module2 (00:00:00)
-
Module-3
Error Handling (Concept) (00:02:21)
Exception Handling1 (00:21:36)
Exception Handling2 (00:31:17)
Exception Handling3 (00:20:53)
Exception Handling Demo (00:09:14)
File Handling (Concept) (00:01:50)
File Handling (00:23:17)
Filehandling-Binaryfiles1 (00:21:50)
File Handling-Binary files2 (00:14:56)
File Handling-Binary Files3 (00:13:19)
Test (00:00:00)
Assignment Module3 (00:00:00)
-
Module-4
OOPs (Concept) (00:01:48)
Abstractions (Concept) (00:01:59)
Abstraction1 (00:30:16)
Abstraction2 (00:19:39)
Abstraction3 (00:18:27)
Abstraction4 (00:26:03)
Interface Demo (00:11:19)
Access Modifiers (00:10:46)
Anonymous Class (00:12:51)
Anonymous class demo (00:06:00)
Construct Overloading (00:33:17)
Constructor (Concept) (00:01:53)
Encapsulation (00:15:45)
Enum1 (00:15:27)
Enum2 (00:16:06)
Enum3 (00:06:01)
This Keyword (Concept) (00:01:49)
Static Keyword (Concept) (00:01:24)
Final Keyword (00:11:49)
Nestedclass1 (00:12:03)
Nestedclass2 (00:12:25)
Nestedclass3 (00:08:11)
Nestedclass4 (00:12:43)
Nestedclass5 (00:05:09)
OOPs Inheritance (00:38:41)
Instanceof (00:14:08)
Non-Accessmodifiers (00:13:01)
Packages (00:07:56)
Polymorphism1 (00:20:55)
Polymorphism2 (00:08:24)
Polymorphism (Concept) (00:01:54)
Singletonclass (00:22:10)
Super Keyword1 (00:12:57)
Super keyword2 (00:09:32)
This Keyword (00:12:06)
Virtual Functions (00:08:20)
Test (00:00:00)
Assignment Module4 (00:00:00)
-
Module-5
Lambda Expression (00:13:26)
Lambda Expression demo (00:05:00)
Threads (Concept) (00:01:36)
Threads1 (00:06:03)
Threads2 (00:36:31)
Threads3 (00:17:28)
Threads4 (00:12:52)
Thread Demo (00:09:10)
Wrapper Classes (00:21:32)
Test (00:00:00)
Assignment Module5 (00:00:00)
-
Module-6
Generic1 (00:12:03)
Generic2 (00:06:25)
Collections1 (00:45:43)
Collections2 (00:11:31)
Collections3 (00:25:40)
Collection4 (00:14:45)
Vectors (00:16:04)
Vector & Thread Demo (00:14:23)
Test (00:00:00)
Assignment Module6 (00:00:00)
-
Module-7
AWT (Concept) (00:01:59)
AWT-GUI-P9 (00:41:28)
Gridbag Layout1 (00:12:06)
Gridbag Layout2 (00:17:54)
AWT-GUI-P2 (00:08:42)
AWT-GUI-P3 (00:41:20)
AWT-GUI-P4 (00:06:42)
AWT-GUI-P5 (00:18:49)
AWT-GUI-P6_2 (00:04:37)
AWT-GUI-P7 (00:45:33)
AWT-GUI-P8 (00:25:51)
Graphics-Draw image (00:01:48)
Graphics-Drawlinerectangle (00:05:40)
Graphics-Drawpoints (00:08:55)
Graphics-Gradientpaint (00:05:52)
Graphics-Texturepaint (00:03:43)
Swings1 (00:36:13)
Swings (Concept) (00:01:50)
JDBCp1 (00:33:04)
JDBCp2 (00:39:06)
Mysql1 (00:18:47)
Mysql2 (00:11:01)
Swing1_1 (00:18:16)
Swings2_1 (00:22:40)
Swings2_2 (00:11:07)
Swings3 (00:18:42)
Swings4 (00:32:46)
Swings5 (00:30:13)
Swings6 (00:08:40)
Swings7 (00:18:13)
Swings8 (00:16:12)
Swings9 (00:06:55)
Swings10 (00:21:27)
Swings11 (00:04:58)
Swings12 (00:12:16)
Swing13 (00:15:15)
Swings14 (00:22:40)
Swings15 (00:06:22)
Swing16 (00:16:14)
Swings17 (00:06:06)
Swings18 (00:13:05)
Swings19 (00:15:56)
Swings20 (00:17:44)
Test (00:00:00)
AWT-GUI-P1 (00:19:46)
GUI_awt_p6 (00:26:00)
Assignment Module7 (00:00:00)
-
Interview Q&A
Interview-1 (00:14:22)
Interview-2 (00:12:11)
Interview-3 (00:21:13)
Interview-4 (00:10:38)
Interview-5 (00:11:39)
Interview-6 (00:24:27)
Interview-7 (00:16:27)
Interview-8 (00:50:01)
Interview-9 (00:10:35)
-
Viva Q&A
Module1 (00:21:12)
Module2 (00:05:51)
Module3 (00:11:45)
Module4 (00:15:41)
Module5 (00:09:06)
Module6 (00:09:00)
Module7 (00:10:50)
-
Project Examples
Example1 (00:24:53)
Example2 (00:36:16)
-
Final Certification Examination
Final Test (00:00:00)
Course Content
Authors
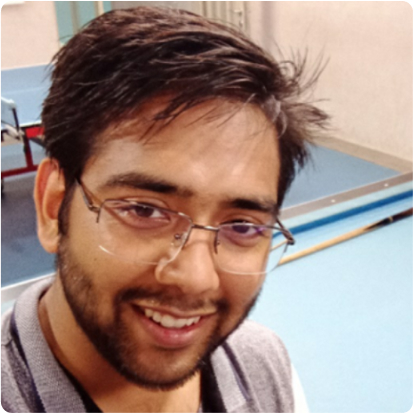
Niteesh Chaudhary
15 DAYS ACCESS
₹0
Start learning today! Switch to full course access, when satisfied with the trial.
PROJECT
Data Modeling with Postgres
In this project, you’ll model user activity data for a music streaming app called Sparkify. You’ll create a relational database and ETL pipeline designed to optimize queries for understanding what songs users are listening to. In PostgreSQL you will also define Fact and Dimension tables and insert data into your new tables.